![[C#] Exception (예외 처리) 핸들링](https://img1.daumcdn.net/thumb/R750x0/?scode=mtistory2&fname=https%3A%2F%2Fblog.kakaocdn.net%2Fdn%2FYOhUq%2FbtsGx1wQYU8%2FK8s0ZZWToLhYrlcQ5a02u1%2Fimg.jpg)
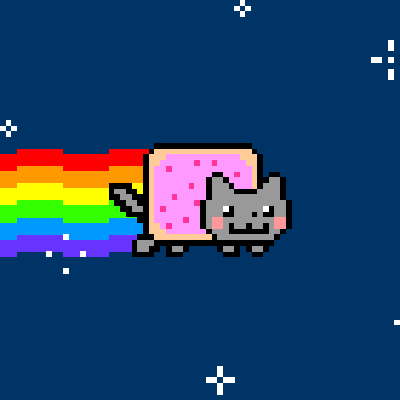
[C#] Exception (예외 처리) 핸들링PROGRAMMING/03. C#2024. 4. 11. 15:16
Table of Contents
반응형
예외 처리란?
컴퓨터 프로그램에서 예외(Excepton)란 프로그램 실행중 예기치 못한 오류가 발생하는 것을 말한다.
평소에는 잘 실행되던 프로그램이 특정한 문제가 발생해서 프로그램이 중단되는 오류이다.
이러한 오류가 발생했을 경우의 처리를 예외처리하고 한다.
- try: 예외 감시자, 예외가 발생할 가능성이 있는 코드의 영역을 지정
- catch: 예외 처리기, try에서 발생한 예외를 확인하고 처리
- finally: 예외가 발생하든 안 하든 무조건 실행시켜야 할 때 사용
- throw: 직접 예외 발생시키기
- when : 특정 예외를 실행하기 위해 처리기에 대해 true여야 하는 조건을 지정하기 위해 catch 절에서 사용
유니티[C#] 예제 - 내가 원하는 예외 처리를 예외 핸들링으로만들어보기
1
2
|
if (obj == null)
throw new IOException("파일을 찾을 수 없음");
|
cs |
원래의 예외처리를 한다면 이렇게 throw문으로 예외를 처리할 수 있다.
IOException 처리를 따로 하지 않아도 일반 예외로 취급이 된다.
1
2
3
4
5
|
catch(IOException e)
{
print(e.Message);
print("파일 탐색 필요");
}
|
cs |
하지만 예외 핸들링(catch)을 통해 내가 원하는 식으로 예외 처리가 가능하다.
예외 처리 전체 코드
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
|
using System;
using System.IO;
using UnityEngine;
public class CharacterObjectNullReferenceException : Exception //Exception으로 부터 상속받음
{
private string selfMessage;
public string SelfMessage
{
get => $"{selfMessage} / {Message}";
}
public override string Message => $"{selfMessage} / {base.Message}"; // 메세지 재정의
private uint code;
public uint Code { get => code; }
public CharacterObjectNullReferenceException()
: base()
{
}
public CharacterObjectNullReferenceException(string message, string selfMessage)
: base(message)
{
this.selfMessage = selfMessage;
}
public CharacterObjectNullReferenceException(string message, string selfMessage, uint code)
: base(message)
{
this.selfMessage = selfMessage;
this.code = code;
}
}
public class Character
{
private GameObject obj;
public Character() // 생성자
{
obj = null;
}
public void Load() // 일방적인 예외
{
if (obj == null)
throw new IOException("파일을 찾을 수 없음");
}
public void Load2() // 원래 예외 객체가 처리할 메세지, 추가메세지
{
if (obj == null)
throw new CharacterObjectNullReferenceException("Referece Not Found", "Monster - Enemy");
}
public void Load3() // 원래 예외 객체가 처리할 메세지, 추가메세지, 코드라인 몇에서 발생한 것인지
{
if (obj == null)
throw new CharacterObjectNullReferenceException("Referece Not Found", "Monster - Enemy", 100);
}
}
public class Test_Exception : MonoBehaviour
{
private Character character;
void Start()
{
character = new Character(); // 무조건 예외 발생
}
private void Update()
{
if(Input.GetKeyDown(KeyCode.Space))
{
try
{
character.Load3();
}
catch(CharacterObjectNullReferenceException e) when(e.Code == 100) //예외 객체의 코드가 100과 같다면 이것으로 처리하게
{
print(e.Message);
print(e.Code);
print("캐릭터 재탐색 후 로드 필요");
}
catch (CharacterObjectNullReferenceException e)
{
print(e.Message);
print("캐릭터 재탐색 후 로드 필요");
}
catch (IOException e)
{
print(e.Message);
print("파일 탐색 필요");
}
}
}
}
|
cs |
출처 및 참고: 강의 짱잘하시는 울 유니티 선생님 수업
반응형
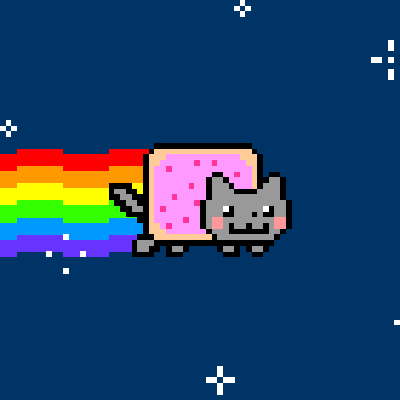
@HYUNJZZANG :: HyunZzang
HyunZzang의 프로그래밍 공간 / 함께 공부해요!!
도움이 되셨다면 "좋아요❤️" 또는 "구독👍🏻" 부탁드립니다 :)