![[C#] SQO / SQL / LinQ](https://img1.daumcdn.net/thumb/R750x0/?scode=mtistory2&fname=https%3A%2F%2Fblog.kakaocdn.net%2Fdn%2FbkgsPu%2FbtsGEqxx0K0%2FnmyuXcKSGIcb8HAzKiFWx0%2Fimg.jpg)
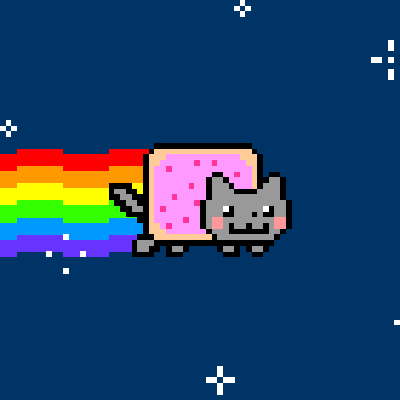
SQO : 표준 질의 연산자
(람다를 이용하여 질의를 편하게 하려는것 [단순한 데이터를 다룰 때])
Structured Query Operator
표준 질의 명령 (표준 요구 명령)
[일단 예제를 보기위해 데이터를 작성해준다 / 하단 코드 참고]
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
|
public class Patent
{
public string Title { get; set; }
public string YearOfPublication { get; set; }
public string ApplicationNumber { get; set; }
public long[] InventorIds { get; set; }
public override string ToString()
{
return $"{ Title } ({ YearOfPublication })";
}
}
public class Inventor
{
public long Id { get; set; }
public string Name { get; set; }
public string City { get; set; }
public string State { get; set; }
public string Country { get; set; }
public override string ToString()
{
return $"{ Name } ({ City }, { State })";
}
}
public static class Datas
{
public static readonly Inventor[] Inventors = new Inventor[]
{
new Inventor()
{
Name = "Benjamin Franklin",
City = "Philadelphia",
State = "PA",
Country = "USA",
Id = 1
},
new Inventor()
{
Name = "Orville Wright",
City = "Kitty Hawk",
State = "NC",
Country = "USA",
Id = 2
},
new Inventor()
{
Name = "Wilbur Wright",
City = "Kitty Hawk",
State = "NC",
Country = "USA",
Id = 3
},
new Inventor()
{
Name = "Samuel Morse",
City = "New York",
State = "NY",
Country = "USA",
Id = 4
},
new Inventor()
{
Name = "George Stephenson",
City = "Wylam",
State = "Northumberland",
Country = "UK",
Id = 5
},
new Inventor()
{
Name = "John Michaelis",
City = "Chicago",
State = "IL",
Country = "USA",
Id = 6
},
new Inventor()
{
Name = "Mary Phelps Jacob",
City = "New York",
State = "NY",
Country = "USA",
Id = 7
},
};
public static readonly Patent[] Patents = new Patent[]
{
new Patent()
{
Title = "Bifocals",
YearOfPublication = "1784",
InventorIds = new long[] { 1 }
},
new Patent()
{
Title = "Phonograph",
YearOfPublication = "1877",
InventorIds = new long[] { 1 }
},
new Patent()
{
Title = "Kinetoscope",
YearOfPublication = "1888",
InventorIds = new long[] { 1 }
},
new Patent()
{
Title = "Electrical Telegraph",
YearOfPublication = "1837",
InventorIds = new long[] { 4 }
},
new Patent()
{
Title = "Flying Machine",
YearOfPublication = "1903",
InventorIds = new long[] { 2, 3 }
},
new Patent()
{
Title = "Steam Locomotive",
YearOfPublication = "1815",
InventorIds = new long[] { 5 }
},
new Patent()
{
Title = "Droplet Deposition Apparatus",
YearOfPublication = "1815",
InventorIds = new long[] { 6 }
},
new Patent()
{
Title = "Backless Brassiere",
YearOfPublication = "1815",
InventorIds = new long[] { 7 }
},
};
}
|
cs |
쿼리명령
⚠️using System.Linq; 를 써줘야 동작한다⚠️
- Select() : 전체 리스트를 보여주는 것
1
|
IEnumerable patents5 = patents.Select(patent => patent.Title);
|
cs |
- Where() : 어떤 조건으로 찾을건지
- StartsWith() : 문자열 시작에 (" ")안이 맞으면 True ["B"가 들어간게 맞다면 리턴을 받아서 IEnumerator에다 넣어준 것]
1
2
3
4
5
|
IEnumerable<string> patents6 =
patents.Select(patent => patent.Title).
Where(str => str.StartsWith("B"));
Print(patents6);
|
cs |
- Count() : 앞의 대한 전체 갯수 리턴
1
2
3
|
int count = patents.Count(patent => patent.Title.StartsWith("B"));
print($"count = {count}"); // 조건에 맞는 것의 수만 출력
print($"Total Count = {patents.Count()}"); // 모든 갯수 출력
|
cs |
- Any() : 해당 요소가 있으면 True, 없으면 False
1
2
|
bool bExist = patents.Any(patent => patent.Title.StartsWith("B")); // 해당 조건이 있느냐
print($"Exsit = {bExist}");
|
cs |
- OrderBy() : 정렬
Patent: 파라미터 / TKey : 리턴타입
1
2
3
4
5
6
7
|
IEnumerable<string> titles = Datas.Patents.Select(patent => patent.Title);
print("--- None Ordered ---");
Print(titles);
IEnumerable<Patent> patents9 = Datas.Patents.OrderBy(patent => patent.Title);
print("--- Ordered ---");
Print(patents9);
|
cs |
Datas.Patents.OrderBy(patent => patent.Title) : 타이틀 기준으로 정렬하는 것
- ThenBy() : 여러가지로 2차 정렬 / 3차 정렬을 하고 싶을 때 (처음엔 Orderby고 이후엔 다 ThenBy 사용)
1
2
3
4
5
6
|
IEnumerable<Patent> patents10 = Datas.Patents.
OrderBy(patent => patent.YearOfPublication).
ThenBy(patent => patent.Title);
print("--- Year Ordered ---");
Print(patents10);
|
cs |
- 자료형 변경
1
2
3
|
// 객체를 생성해서 배열식으로 리턴하는 것도 가능하다
IEnumerable<FileInfo> fileInfo = files.Select(file => new FileInfo(file));
FileInfo[] fileInfos2 = files.Select(file => new FileInfo(file)).ToArray();
|
cs |
- var을 사용하여 익명으로 원하는 자료형만 뽑아서 리턴받기
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
// 익명으로 리턴
var fileItems = fileInfos.Select
(
file =>
{
return new
{
FileName = file.Name,
FullName = file.FullName,
Size = file.Length,
Data = file.CreationTime, // 날짜를 다룸
};
}
);
Print(fileItems);
|
cs |
- OfType() : 해당 타입에 맞는 것만 리턴
1
2
3
4
5
6
7
8
9
|
IEnumerable<object> stuff = new object[]
{
1, 3, new object(), 5, 7, 9, "\"name\"", Guid.NewGuid(), 11
};
IEnumerable<int> even = new int[] { 0, 2, 4, 6, 8, 10 };
IEnumerable<int> odd = stuff.OfType<int>();
Print(odd);
|
cs |
- Union() : 2개의 데이터를 하나의 배열로 나타내주는데, 중복되는 건 제거한다 (같은 건 X)
1
2
3
|
IEnumerable<int> union = even.Union(odd);
print("-- Union");
Print(union);
|
cs |
- Concat() : 2개의 데이터를 하나의 배열로 나타내주는데, 중복을 허용해준다 (같은게 있어도 O)
1
2
3
|
IEnumerable<int> concat = even.Concat(odd);
print("-- Concat");
Print(concat);
|
cs |
- Distinct() : 중복 제거 / 따라서 Concat에 Distinct()를 붙이면 결과는 유니온과 동일하다
1
|
IEnumerable<int> concat2 = even.Concat(odd).OrderBy(value => value).Distinct();
|
cs |
- Reverse() : 거꾸로 출력
- Count() : 수
- Sum() : 합계
- Average() : 평균
- Max() : 최대값
- Min() : 최소값
⚠️숫자 자료형만 가능⚠️
1
2
3
4
5
|
print($"Count = {numbers.Count()}");
print($"Sum = {numbers.Sum()}");
print($"Average = {numbers.Average()}");
print($"Max = {numbers.Max()}");
print($"Min = {numbers.Min()}");
|
cs |
LinQ
(SQO를 문자열로 다루는 것 [복잡한 데이터를 다룰 때])
Language Integrated Query 라고해서 특정 데이터들에서 Query를 하여 데이터를 빠르고 편리하게 추출하는 방식이라 할 수 있다. 기본적으로 람다표현식을 사용하여 간결하고 가독성 좋게 작성 가능하다. Query를 하는데에는 SQL을 사용한다. SQL 이란 Structured Query Language의 약자이다.
- from : 어떤 데이터에서 찾을 것인가
- where : 어떤 조건으로 찾을 것인가
- order by : 어떤 항목을 기준으로 정렬할 것인가
- select : 어떤 항목을 추출할 것인가
1
|
IEnumerable<Patent> patents = from patent in Datas.Patents select patent;
|
cs |
- in Datas.Patents : 어떤 배열에서 가져올것인지
- from patent : 어떤 변수로 다룰것인지
- select patent 리턴할 요소
- where
: Title이 B로 시작하는 것들 찾기
1
2
3
4
5
6
|
IEnumerable<Patent> patents3 =
from patent in Datas.Patents
where patent.Title.StartsWith("B")
select patent;
Print(patents3);
|
cs |
- order by
: ~을 기준으로 정렬
1
2
3
4
5
6
|
IEnumerable<Patent> patents4 =
from patent in Datas.Patents
orderby patent.Title
select patent;
Print(patents4);
|
cs |
- orderby로 2차(이중) 정렬
: (descending: 내림차순 / asceding: 오름차순)
1
2
3
4
5
6
|
IEnumerable<Patent> patents5 =
from patent in Datas.Patents
orderby patent.YearOfPublication descending, patent.Title ascending // 내림차순, 오름차순
select patent;
Print(patents5);
|
cs |
- 익명형식 (var) 사용
1
2
3
4
5
6
7
8
9
10
11
|
var fileItems =
from file in fileInfos
select new
{
FileName = file.Name,
FullName = file.FullName,
Size = file.Length,
Data = file.CreationTime
};
Print(fileItems);
|
cs |
출처 및 참고: 강의 짱잘하시는 울 유니티 선생님 수업
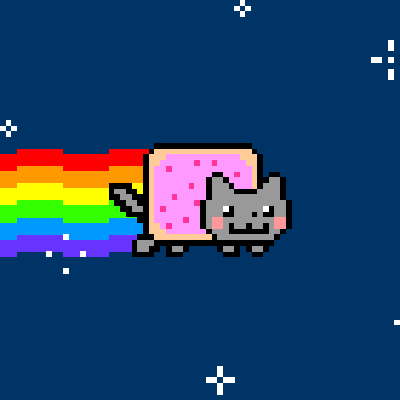
HyunZzang의 배우는 공간 / 함께 공부해요!!
도움이 되셨다면 "좋아요❤️" 또는 "구독👍🏻" 부탁드립니다 :)