![[C#] StreamWriter와 StreamReader](https://img1.daumcdn.net/thumb/R750x0/?scode=mtistory2&fname=https%3A%2F%2Fblog.kakaocdn.net%2Fdn%2Fb9UAbx%2FbtsGuIEWZ6s%2FMEk3b4dl48PqJsUPQHBvk1%2Fimg.jpg)
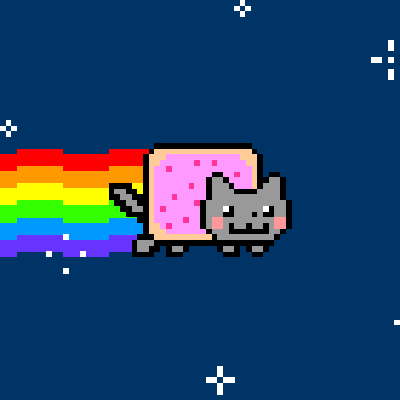
StreamWriter와 StreamReader
우리는 C#에서 파일을 읽고 쓰기 위한 스트림으로 StreamReader와 StreamWriter를 사용할 수 있다.
File 클래스도 있긴 하지만 읽고 쓸 땐 StreamReader와 StreamWriter을 더 많이 사용한다
이 클래스들은 System.IO 네임스페이스에 선언되어 있으므로 사용하기 위해서는 System.IO를 using 해줘야 한다.
(C# - 유니티예제) Stream Writer : 파일 쓰기
( ) 부분에는 파일에 대한 절대 경로가 들어간다.
WriteLine은 한 줄씩 쓰는 것이다.
숫자도 가능하다.
Stream Writer는 열었으면 무조건 닫아줘야 한다. 따라서 Close()를 반드시 콜 해줘야 한다. 그렇게 하지 않으면, 파일이 열린 상태로 종료되기 때문에, 그 파일을 다시 열라고 하면 '이 파일은 다른 프로그램에서 열려있습니다' 이런 경고가 뜬다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
|
private void OnGUI()
{
GUILayout.BeginVertical();
{
if (GUILayout.Button("Save_TextFile"))
Save_TextFile();
}
GUILayout.EndVertical();
}
private void Save_TextFile()
{
string directory = Application.dataPath + "/UnitTests/Test_TextFile/";
string path = EditorUtility.SaveFilePanel("Save TextFile", directory, "Text", "txt");
if (path.Length < 1)
return;
StreamWriter writer = new StreamWriter(path); // ()에는 파일에 대한 절대 경로가 들어간다
{
writer.WriteLine("Line 1");
writer.WriteLine("Line 2");
int value = 10;
writer.WriteLine(value);
}
writer.Close();
}
|
cs |
이렇게 코드를 작성 한 다음 실행을 해 보면
게임 화면 위에 Save_TextFile이라는 버튼이 떠 있다. 버튼을 눌러보면
이렇게 파일 저장창이 뜬다. 저장을 해보면
이렇게 Assets에 텍스트 파일이 생성된 걸 볼 수 있다.
(C# - 유니티예제) Stream Reader : 파일 읽기
Stream Writer와 같이 Close()를 꼭 써준다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
|
private void Read_TextFile()
{
string directory = Application.dataPath + "/UnitTests/Test_TextFile/";
string path = EditorUtility.OpenFilePanel("Save TextFile", directory, "txt");
if (path.Length < 1)
return;
StreamReader reader = new StreamReader(path);
{
string line;
while((line = reader.ReadLine()) != null) // ReadLine : 한 줄씩 읽어주는 것
{
print(line);
}
}
reader.Close();
}
|
cs |
1
2
3
4
5
6
7
8
9
10
11
12
|
private void OnGUI()
{
GUILayout.BeginVertical();
{
if (GUILayout.Button("Save_TextFile"))
Save_TextFile();
if (GUILayout.Button("Read_TextFile"))
Read_TextFile();
}
GUILayout.EndVertical();
}
|
cs |
아까 생성한 Text.txt 파일을 열어보자.
콘솔창에 방금 썼던 txt 파일이 읽히는 모습을 볼 수 있다.
(C# - 유니티예제) Stream Writer : CSV 파일 쓰기
CSV(comma-separated values)는 몇 가지 필드를 쉼표(,)로 구분한 텍스트 데이터 및 텍스트 파일이다. 확장자는 .csv이며 MIME 형식은 text/csv이다. (행은 엔터로, 라인은 콤마로 구분)
일단 필요한 아이템 데이터를 구조체로 만들어 놓는다.
1
2
3
4
5
6
7
|
private struct ItemData
{
public int Id;
public string Name;
public float Recovery;
public float Ratio;
}
|
cs |
그리고 Csv 파일을 읽기 위한 함수도 만들어준다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
|
private void Save_CsvFile()
{
string directory = Application.dataPath + "/UnitTests/Test_TextFile/";
string path = EditorUtility.SaveFilePanel("Save CsvFile", directory, "Test", "csv");
if (path.Length < 1)
return;
ItemData[] datas = new ItemData[4]; // 아이템 배열 생성
//아이템 0번
{
datas[0].Id = 0x00000001;
datas[0].Name = "Position";
datas[0].Recovery = 20.0f;
datas[0].Ratio = 0.5f;
}
//1
{
datas[1].Id = 0x00000002;
datas[1].Name = "Big Position";
datas[1].Recovery = 50.0f;
datas[1].Ratio = 0.8f;
}
//2
{
datas[2].Id = 0x00000003;
datas[2].Name = "Poison Position";
datas[2].Recovery = -10.0f;
datas[2].Ratio = 1.0f;
}
//3
{
datas[3].Id = 0x00000004;
datas[3].Name = "Mana Position";
datas[3].Recovery = 60.0f;
datas[3].Ratio = -1.0f;
}
StreamWriter writer = new StreamWriter(path); // ()에는 파일에 대한 절대 경로가 들어간다
{
foreach(ItemData data in datas)
{
writer.Write(data.Id + ",");
writer.Write(data.Name + ",");
writer.Write(data.Recovery + ",");
writer.Write(data.Ratio);
writer.WriteLine();
}
}
writer.Close();
}
|
cs |
생성된 파일을 엑셀이나 메모장에 끌어다 놓으면, 이렇게 나타난다.
(C# - 유니티예제) Stream Writer : CSV 파일 읽기
읽는 것도 Txt 파일과 유사하다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
|
private void Read_CsvFile()
{
string directory = Application.dataPath + "/UnitTests/Test_TextFile/";
string path = EditorUtility.OpenFilePanel("Read CsvFile", directory, "csv");
if (path.Length < 1)
return;
StreamReader reader = new StreamReader(path);
{
string line;
while ((line = reader.ReadLine()) != null) // ReadLine : 한 줄씩 읽어주는 것
{
string[] str = line.Split(','); // 한 줄이 콤마로 구분 되어있으니 콤마로 짤라준다 -> 그것을 배열로 생성
for (int i = 0; i < str.Length; i++)
print(str[i]);
}
}
reader.Close();
}
|
cs |
이렇게 내가 작성했던 CSV 파일을 읽어들이는 모습을 볼 수 있다.
출처 및 참고: 강의 짱잘하시는 울 유니티 선생님 수업
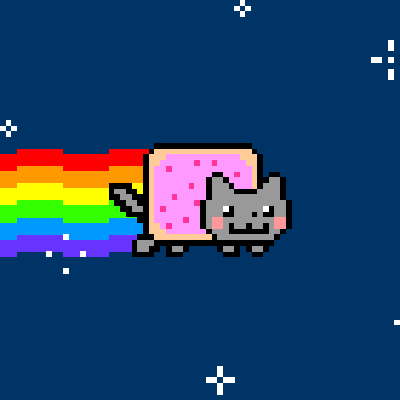
HyunZzang의 배우는 공간 / 함께 공부해요!!
도움이 되셨다면 "좋아요❤️" 또는 "구독👍🏻" 부탁드립니다 :)