![[C#] 연산자 오버로딩](https://img1.daumcdn.net/thumb/R750x0/?scode=mtistory2&fname=https%3A%2F%2Fblog.kakaocdn.net%2Fdn%2FezS95H%2FbtsGB48Kj1p%2FM0rD5IqQkiilg8K9XvdkZk%2Fimg.jpg)
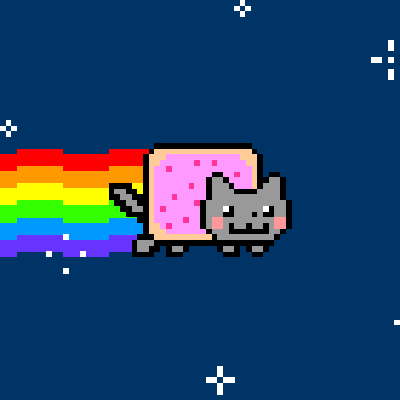
[C#] 연산자 오버로딩PROGRAMMING/03. C#2024. 4. 15. 10:43
Table of Contents
반응형
연산자 오버로딩
C# 에서는 사용자 정의 (Class / Struct) 형식에서 operator 키워드로 정적 멤버 함수를 정의하여 연산자를 오버로드 할 수 있다. 모든 연산자를 오버로드할 수 있는 것은 아니며, 일부 제한이 있는 연산자도 있다.
➡️연산자 오버로딩 - 단항, 산술, 항등 및 비교 연산자를 정의합니다. - C# | Microsoft Learn
Equals() 재정의
유니티[C#] - 예제
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
|
Coordinate coordinate = new Coordinate()
{
X = new Vector3(10, 20, 30), // public 일 때 변수초기화
Y = new Vector3(20, 30, 40),
};
Coordinate coordinate2 = new Coordinate()
{
X = new Vector3(10, 20, 30), // public 일 때 변수초기화
Y = new Vector3(20, 30, 40),
};
Coordinate coordinate3 = new Coordinate()
{
X = new Vector3(20, 20, 30), // public 일 때 변수초기화
Y = new Vector3(30, 30, 40),
};
Coordinate coordinate4 = coordinate;
print($"coorinate == coorinate2 : {coordinate.Equals(coordinate2)}"); // True
print($"coorinate == coorinate3 : {coordinate.Equals(coordinate3)}"); // False
print($"coorinate == coorinate4 : {coordinate.Equals(coordinate4)}"); // True : 애초에 참조주소가 같기 때문에
|
cs |
비교 연산자 [ ==(같다) / !=(같지않다 ] 재정의
주의할 점 : 비교 연산자를 오버로드하는 경우에는 쌍으로 오버로드 해야 함 (== 연산자를 오버로드하면 != 연산자도 오버로드 해야 됨)
유니티[C#] - 예제
1
2
3
4
5
6
7
8
9
10
|
public static bool operator ==(Coordinate lhs, Coordinate rhs)
{
return lhs.Equals(rhs);
}
public static bool operator !=(Coordinate lhs, Coordinate rhs)
{
return !(lhs == rhs);
}
|
cs |
이항 연산자 (+, -, *, /, %, &, |, ^, <<, >>) 재정의
유니티[C#] - +이항연산자 예제
1
2
3
4
|
public static Coordinate operator +(Coordinate lhs, Coordinate rhs)
{
return new Coordinate(lhs.X + rhs.X, lhs.Y + rhs.Y);
}
|
cs |
1
2
3
4
5
6
7
8
9
10
11
|
Coordinate coordinate5 = new Coordinate()
{
X = new Vector3(20, 20, 30),
Y = new Vector3(30, 30, 40),
};
Coordinate coordinate6 = coordinate + coordinate5;
Coordinate coordinate7 = coordinate + 5.0f;
print($"coordinate6 : {coordinate6}");
print($"coordinate7 : {coordinate7}");
|
cs |
단항연산자 ( +, -, !, ~, ++, --, true, false) 재정의
유니티[C#] -단항연산자 예제
1
2
3
4
|
public static Coordinate operator -(Coordinate sh)
{
return new Coordinate(-sh.X, -sh.Y);
}
|
cs |
C# 단항연산자 ++ 예제
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
|
using System;
class EvenNum
{
int evenNumber;
public EvenNum(int n)
{
evenNumber = (n % 2 == 0) ? n : n + 1;
}
public static EvenNum operator++(EvenNum e)
{
e.evenNumber += 2;
return e;
}
public static EvenNum operator--(EvenNum e)
{
e.evenNumber -= 2;
return e;
}
public void PrintEven()
{
Console.WriteLine($"Even Number = {evenNumber}");
}
}
class OperatorOverloadingApp
{
public static void Main()
{
EvenNum e = new EvenNum(4);
e.PrintEven();
e++;
e.PrintEven();
e--;
e.PrintEven();
}
}
|
cs |
캐스팅 연산자 (explicit, Implicit) 재정의
유니티[C#] - 명시적 변환 예제
- 명시적 변환 (explicit)
- 단항만 들어와야 한다
1
2
3
4
|
public static explicit operator float(Coordinate sh)
{
return Vector3.Dot(sh.X, sh.Y); // 곱한 것
}
|
cs |
1
2
|
float temp = (float)coordinate7; // 암시적으로 (float)을 써 주지 않으면 오류 발생
print($"temp = {temp}");
|
cs |
Indexer
배열처럼 []를 통해 클래스의 요소에 접근할 수 있도록 제공되는 구문이다.
(C#은 배열을 재정의 할 수 없지만 indexer를 제공해준다)
유니티[C#] - Indexer 예제
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
|
private class NumberCollection<T>
{
private T[] array = new T[10];
public T this[int i]
{
get { return array[i]; }
set { array[i] = value; }
}
public int Length
{
get { return array.Length; }
}
}
void Start()
{
NumberCollection<int> number = new NumberCollection<int>();
for (int i = 0; i < number.Length; i++)
number[i] = Random.Range(0, 20);
for (int i = 0; i < number.Length; i++)
print($"number[{i}] = {number[i]}");
}
|
cs |
출처 및 참고: 강의 짱잘하시는 울 유니티 선생님 수업
반응형
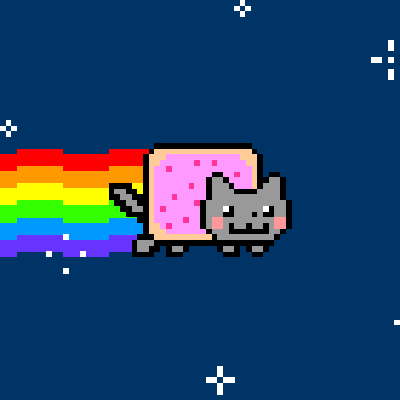
@HYUNJZZANG :: HyunZzang
HyunZzang의 프로그래밍 공간 / 함께 공부해요!!
도움이 되셨다면 "좋아요❤️" 또는 "구독👍🏻" 부탁드립니다 :)